How Do I Run the if Statement Again if User Pressed Wrong Choice in Python

Welcome! If you want to acquire how to piece of work with while loops in Python, so this article is for you.
While loops are very powerful programming structures that you tin can apply in your programs to repeat a sequence of statements.
In this article, yous will learn:
- What while loops are.
- What they are used for.
- When they should be used.
- How they work behind the scenes.
- How to write a while loop in Python.
- What infinite loops are and how to interrupt them.
- What
while True
is used for and its general syntax. - How to use a
break
statement to terminate a while loop.
You will acquire how while loops work backside the scenes with examples, tables, and diagrams.
Are you gear up? Let'due south begin. 🔅
🔹 Purpose and Use Cases for While Loops
Let's starting time with the purpose of while loops. What are they used for?
They are used to repeat a sequence of statements an unknown number of times. This type of loop runs while a given condition is True
and it only stops when the status becomes Fake
.
When we write a while loop, we don't explicitly ascertain how many iterations will be completed, we just write the condition that has to be True
to continue the process and False
to stop it.
💡 Tip: if the while loop condition never evaluates to Fake
, then we will have an space loop, which is a loop that never stops (in theory) without external intervention.
These are some examples of real use cases of while loops:
- User Input: When we inquire for user input, nosotros demand to check if the value entered is valid. We can't perhaps know in accelerate how many times the user will enter an invalid input earlier the programme tin continue. Therefore, a while loop would be perfect for this scenario.
- Search: searching for an element in a data structure is some other perfect use case for a while loop because we can't know in advance how many iterations will be needed to find the target value. For example, the Binary Search algorithm tin can be implemented using a while loop.
- Games: In a game, a while loop could be used to keep the main logic of the game running until the player loses or the game ends. Nosotros tin't know in advance when this volition happen, and so this is some other perfect scenario for a while loop.
🔸 How While Loops Work
At present that you lot know what while loops are used for, let'due south encounter their main logic and how they work behind the scenes. Here we accept a diagram:

Let'southward break this down in more detail:
- The procedure starts when a while loop is found during the execution of the plan.
- The condition is evaluated to check if information technology'southward
True
orFalse
. - If the condition is
Truthful
, the statements that belong to the loop are executed. - The while loop condition is checked again.
- If the condition evaluates to
Truthful
again, the sequence of statements runs again and the procedure is repeated. - When the condition evaluates to
False
, the loop stops and the program continues across the loop.
One of the most important characteristics of while loops is that the variables used in the loop condition are non updated automatically. We take to update their values explicitly with our code to make sure that the loop will eventually end when the condition evaluates to Fake
.
🔹 General Syntax of While Loops
Peachy. Now you know how while loops piece of work, so let's swoop into the code and meet how you lot can write a while loop in Python. This is the basic syntax:

These are the main elements (in gild):
- The
while
keyword (followed by a space). - A condition to determine if the loop volition go on running or not based on its truth value (
True
orImitation
). - A colon (
:
) at the end of the first line. - The sequence of statements that will be repeated. This cake of code is called the "body" of the loop and it has to be indented. If a statement is not indented, it will not be considered part of the loop (delight see the diagram below).
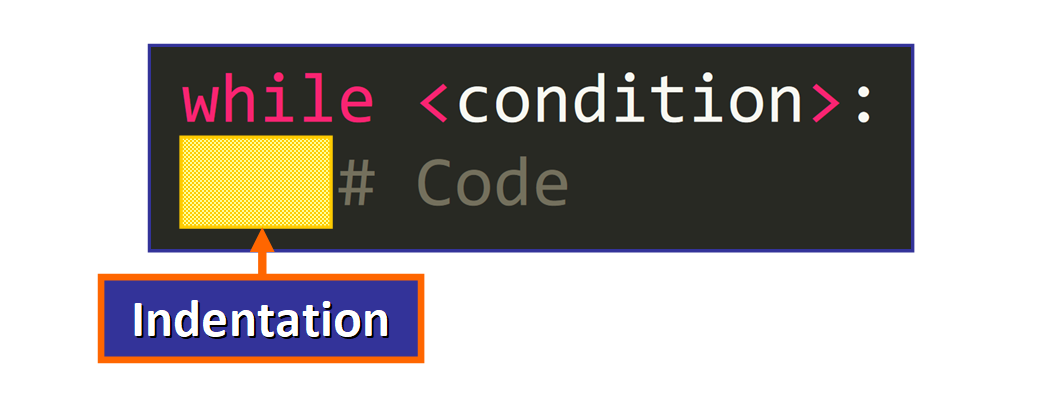
💡 Tip: The Python manner guide (PEP 8) recommends using 4 spaces per indentation level. Tabs should just be used to remain consistent with code that is already indented with tabs.
🔸 Examples of While Loops
Now that you know how while loops work and how to write them in Python, let'due south see how they work backside the scenes with some examples.
How a Basic While Loop Works
Here we have a basic while loop that prints the value of i
while i
is less than eight (i < 8
):
i = four while i < eight: print(i) i += 1
If we run the code, we see this output:
4 five 6 7
Let's run into what happens behind the scenes when the code runs:
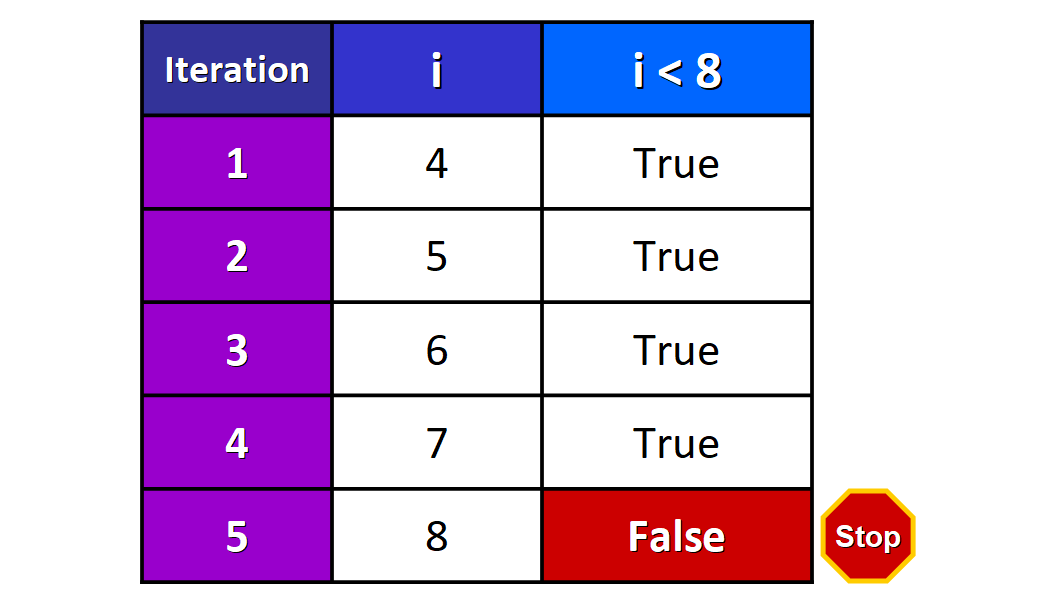
- Iteration one: initially, the value of
i
is 4, then the conditioni < 8
evaluates toTruthful
and the loop starts to run. The value ofi
is printed (four) and this value is incremented by 1. The loop starts again. - Iteration 2: now the value of
i
is v, so the conditioni < eight
evaluates toTrue
. The torso of the loop runs, the value ofi
is printed (5) and this valuei
is incremented by i. The loop starts once again. - Iterations 3 and 4: The same procedure is repeated for the tertiary and fourth iterations, so the integers 6 and seven are printed.
- Before starting the fifth iteration, the value of
i
isviii
. Now the while loop conditioni < 8
evaluates toFalse
and the loop stops immediately.
💡 Tip: If the while loop condition is False
before starting the first iteration, the while loop will not even commencement running.
User Input Using a While Loop
Now let'south see an example of a while loop in a program that takes user input. We will the input()
function to ask the user to enter an integer and that integer volition simply be appended to list if information technology'southward even.
This is the code:
# Define the list nums = [] # The loop volition run while the length of the # list nums is less than 4 while len(nums) < 4: # Ask for user input and store it in a variable as an integer. user_input = int(input("Enter an integer: ")) # If the input is an even number, add information technology to the listing if user_input % 2 == 0: nums.suspend(user_input)
The loop status is len(nums) < 4
, so the loop will run while the length of the listing nums
is strictly less than iv.
Let's analyze this plan line by line:
- Nosotros start past defining an empty list and assigning information technology to a variable chosen
nums
.
nums = []
- Then, we define a while loop that will run while
len(nums) < 4
.
while len(nums) < four:
- Nosotros inquire for user input with the
input()
function and store information technology in theuser_input
variable.
user_input = int(input("Enter an integer: "))
💡 Tip: Nosotros demand to catechumen (cast) the value entered by the user to an integer using the int()
role before assigning it to the variable considering the input()
function returns a string (source).
- Nosotros bank check if this value is even or odd.
if user_input % 2 == 0:
- If it'southward fifty-fifty, we append it to the
nums
listing.
nums.append(user_input)
- Else, if information technology'southward odd, the loop starts again and the condition is checked to determine if the loop should keep or not.
If nosotros run this code with custom user input, we get the post-obit output:
Enter an integer: 3 Enter an integer: 4 Enter an integer: two Enter an integer: 1 Enter an integer: 7 Enter an integer: vi Enter an integer: three Enter an integer: 4
This table summarizes what happens behind the scenes when the code runs:

💡 Tip: The initial value of len(nums)
is 0
because the list is initially empty. The terminal column of the table shows the length of the listing at the end of the current iteration. This value is used to bank check the condition earlier the next iteration starts.
As you can encounter in the table, the user enters even integers in the 2nd, third, sixth, and eight iterations and these values are appended to the nums
listing.
Before a "ninth" iteration starts, the condition is checked again but now information technology evaluates to False
because the nums
list has four elements (length iv), and then the loop stops.
If we cheque the value of the nums
listing when the procedure has been completed, we see this:
>>> nums [iv, 2, six, 4]
Exactly what we expected, the while loop stopped when the condition len(nums) < 4
evaluated to False
.
Now you know how while loops work behind the scenes and y'all've seen some practical examples, and so let'due south dive into a cardinal chemical element of while loops: the condition.
🔹 Tips for the Condition in While Loops
Before you lot start working with while loops, you should know that the loop status plays a fundamental role in the functionality and output of a while loop.

You must be very conscientious with the comparing operator that you choose because this is a very common source of bugs.
For example, common errors include:
- Using
<
(less than) instead of<=
(less than or equal to) (or vice versa). - Using
>
(greater than) instead of>=
(greater than or equal to) (or vice versa).
This can touch on the number of iterations of the loop and even its output.
Let'south see an example:
If we write this while loop with the condition i < 9
:
i = 6 while i < 9: print(i) i += i
We run across this output when the lawmaking runs:
half dozen vii 8
The loop completes three iterations and information technology stops when i
is equal to ix
.
This table illustrates what happens behind the scenes when the lawmaking runs:
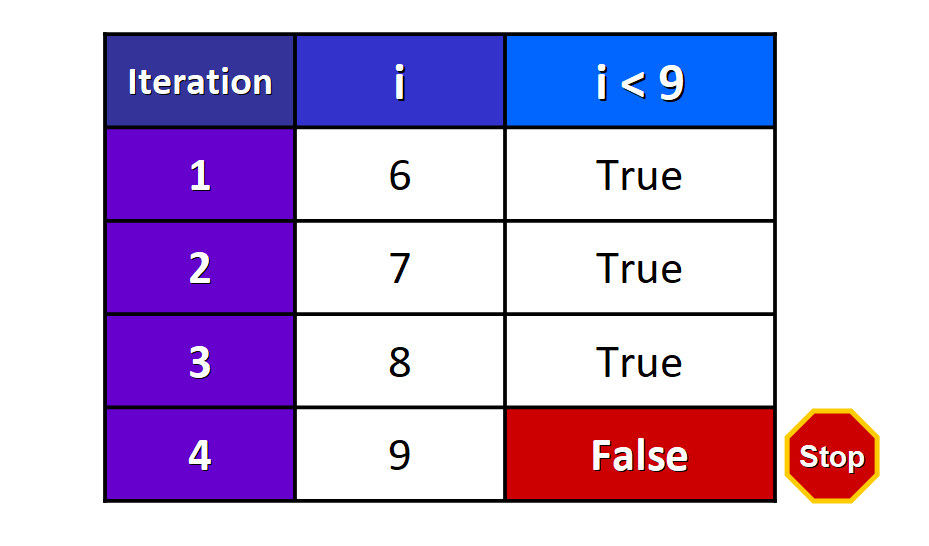
- Before the commencement iteration of the loop, the value of
i
is vi, so the conditioni < 9
isTrue
and the loop starts running. The value ofi
is printed and and so information technology is incremented by one. - In the 2nd iteration of the loop, the value of
i
is 7, so the statusi < 9
isTrue
. The trunk of the loop runs, the value ofi
is printed, and and then it is incremented past 1. - In the third iteration of the loop, the value of
i
is 8, so the conditioni < 9
isTrue
. The body of the loop runs, the value ofi
is printed, and then information technology is incremented by ane. - The condition is checked again before a quaternary iteration starts, merely now the value of
i
is nine, soi < 9
isFalse
and the loop stops.
In this case, we used <
every bit the comparison operator in the status, but what practise you think will happen if nosotros use <=
instead?
i = half-dozen while i <= 9: print(i) i += one
We run across this output:
half-dozen seven 8 9
The loop completes one more iteration because at present we are using the "less than or equal to" operator <=
, and then the condition is still Truthful
when i
is equal to nine
.
This table illustrates what happens behind the scenes:

Four iterations are completed. The condition is checked again earlier starting a "fifth" iteration. At this point, the value of i
is 10
, so the condition i <= 9
is Imitation
and the loop stops.
🔸 Space While Loops
At present you know how while loops work, but what practice you think will happen if the while loop condition never evaluates to False
?
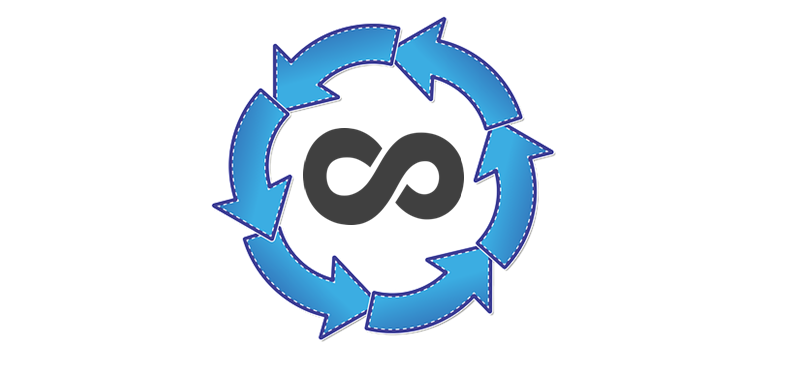
What are Space While Loops?
Remember that while loops don't update variables automatically (nosotros are in charge of doing that explicitly with our lawmaking). So in that location is no guarantee that the loop volition cease unless we write the necessary code to make the condition False
at some signal during the execution of the loop.
If nosotros don't do this and the condition always evaluates to True
, so nosotros will accept an infinite loop, which is a while loop that runs indefinitely (in theory).
Space loops are typically the consequence of a problems, only they tin besides be caused intentionally when we want to repeat a sequence of statements indefinitely until a break
statement is found.
Permit's come across these two types of infinite loops in the examples below.
💡 Tip: A bug is an error in the program that causes incorrect or unexpected results.
Example of Infinite Loop
This is an instance of an unintentional infinite loop caused by a problems in the program:
# Ascertain a variable i = v # Run this loop while i is less than 15 while i < 15: # Print a bulletin print("Hullo, World!")
Analyze this code for a moment.
Don't you lot notice something missing in the torso of the loop?
That'due south correct!
The value of the variable i
is never updated (information technology's always 5
). Therefore, the condition i < fifteen
is ever True
and the loop never stops.
If we run this code, the output volition be an "infinite" sequence of Howdy, World!
letters because the body of the loop impress("Hello, World!")
will run indefinitely.
Hello, World! Hullo, World! How-do-you-do, World! Hello, Globe! How-do-you-do, World! Hello, World! Hello, World! How-do-you-do, Earth! Hi, Globe! How-do-you-do, World! Hello, World! Hello, World! Hello, World! Howdy, Earth! Hello, World! Hi, Earth! Hello, World! Hello, World! . . . # Continues indefinitely
To stop the plan, we volition need to interrupt the loop manually past pressing CTRL + C
.
When we do, we will come across a KeyboardInterrupt
fault like to this one:

To fix this loop, we will need to update the value of i
in the trunk of the loop to make sure that the condition i < 15
will eventually evaluate to Faux
.
This is one possible solution, incrementing the value of i
by 2 on every iteration:
i = 5 while i < 15: print("Hullo, Earth!") # Update the value of i i += ii
Nifty. Now you know how to ready space loops acquired by a bug. Yous just need to write code to guarantee that the status volition somewhen evaluate to False
.
Allow'due south first diving into intentional space loops and how they piece of work.
🔹 How to Make an Space Loop with While True
We tin generate an infinite loop intentionally using while True
. In this case, the loop will run indefinitely until the process is stopped by external intervention (CTRL + C
) or when a pause
statement is found (you will learn more nearly break
in just a moment).
This is the basic syntax:

Instead of writing a status after the while
keyword, we simply write the truth value directly to bespeak that the status will ever be True
.
Here we have an case:
>>> while True: print(0) 0 0 0 0 0 0 0 0 0 0 0 0 0 Traceback (most contempo call last): File "<pyshell#two>", line 2, in <module> impress(0) KeyboardInterrupt
The loop runs until CTRL + C
is pressed, merely Python also has a break
argument that we can employ directly in our lawmaking to stop this blazon of loop.
The suspension
statement
This statement is used to cease a loop immediately. Yous should think of information technology as a red "stop sign" that you tin can apply in your code to accept more command over the behavior of the loop.

Co-ordinate to the Python Documentation:
Theinterruption
argument, like in C, breaks out of the innermost enclosingfor
orwhile
loop.
This diagram illustrates the basic logic of the suspension
statement:

interruption
statement This is the basic logic of the break
statement:
- The while loop starts merely if the condition evaluates to
Truthful
. - If a
break
statement is found at any indicate during the execution of the loop, the loop stops immediately. - Else, if
break
is not establish, the loop continues its normal execution and it stops when the status evaluates toFalse
.
We can use break
to end a while loop when a condition is met at a particular point of its execution, and so you will typically observe information technology within a conditional statement, like this:
while Truthful: # Code if <status>: break # Code
This stops the loop immediately if the status is True
.
💡 Tip: You tin (in theory) write a break
statement anywhere in the trunk of the loop. Information technology doesn't necessarily have to be part of a conditional, just we commonly use it to stop the loop when a given condition is Truthful
.
Here we have an case of break
in a while Truthful
loop:
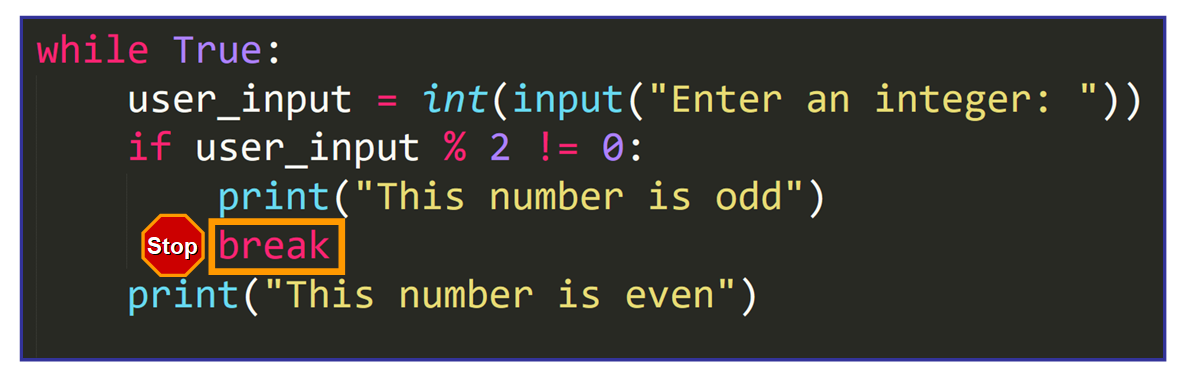
Let's see it in more than detail:
The first line defines a while True
loop that will run indefinitely until a intermission
argument is constitute (or until it is interrupted with CTRL + C
).
while Truthful:
The second line asks for user input. This input is converted to an integer and assigned to the variable user_input
.
user_input = int(input("Enter an integer: "))
The third line checks if the input is odd.
if user_input % 2 != 0:
If information technology is, the message This number is odd
is printed and the break
statement stops the loop immediately.
print("This number of odd") break
Else, if the input is even , the bulletin This number is even
is printed and the loop starts once more.
impress("This number is even")
The loop will run indefinitely until an odd integer is entered because that is the only way in which the break
statement will be establish.
Here we have an example with custom user input:
Enter an integer: four This number is even Enter an integer: half-dozen This number is fifty-fifty Enter an integer: 8 This number is even Enter an integer: 3 This number is odd >>>
🔸 In Summary
- While loops are programming structures used to repeat a sequence of statements while a condition is
True
. They stop when the condition evaluates toFake
. - When you write a while loop, you lot need to make the necessary updates in your lawmaking to brand certain that the loop will eventually stop.
- An infinite loop is a loop that runs indefinitely and it only stops with external intervention or when a
break
statement is found. - Yous tin can stop an infinite loop with
CTRL + C
. - You tin generate an infinite loop intentionally with
while True
. - The
intermission
argument can exist used to end a while loop immediately.
I really hope you liked my article and found information technology helpful. Now you know how to work with While Loops in Python.
Follow me on Twitter @EstefaniaCassN and if you desire to learn more about this topic, check out my online course Python Loops and Looping Techniques: Beginner to Advanced.
Acquire to code for free. freeCodeCamp's open source curriculum has helped more than than twoscore,000 people go jobs every bit developers. Get started
Source: https://www.freecodecamp.org/news/python-while-loop-tutorial/
0 Response to "How Do I Run the if Statement Again if User Pressed Wrong Choice in Python"
Post a Comment